An Application Programming Interface (API) is a set of rules and protocols that specifies how two software programs should interact with each other. It serves as an intermediary between different software systems, allowing them to communicate and exchange information with each other.
An API defines a set of functions that a developer can use in their own application to access the features or data of an operating system, application, or other service. For example, the Google Maps API allows a developer to include Google Maps in their own web application, and the Twitter API allows a developer to access and retrieve data from Twitter’s servers.
APIs can be classified into different types, such as:
- Web-based APIs: These APIs can be accessed over the internet using standard protocols like HTTP or HTTPS.
- Operating system APIs: These APIs provide access to the features and functionality of an operating system, such as reading and writing files, starting and stopping processes, and displaying notifications.
- Library APIs: These APIs provide access to a set of pre-built software components or libraries that can be used in an application to perform common tasks, such as data parsing, encryption, or image manipulation.
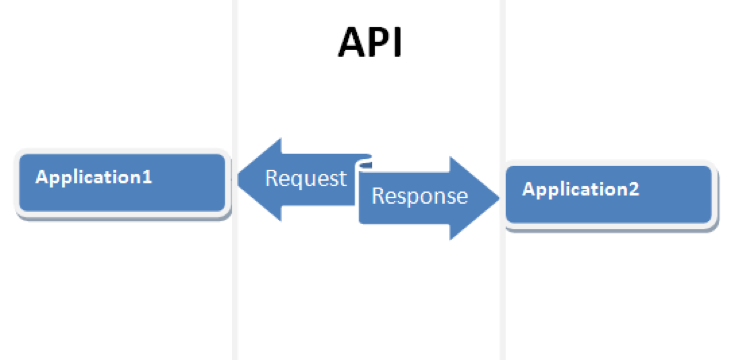
In this example, an application (App 1) makes a request to a server using an API. The server processes the request and returns the data or functionality requested by the application.
Here are a few examples of real-world case studies that demonstrate the use of APIs:
- Facebook Login: Facebook’s API allows developers to integrate Facebook Login into their own web and mobile applications. This allows users to log in to the third-party application using their Facebook credentials, and share their data and activity with the application.
- Uber API: The Uber API allows developers to request and retrieve data from Uber’s platform, including information about drivers, vehicles, and rides. This allows developers to build applications that can request rides, track the progress of a ride, and rate the driver, all without having to directly access Uber’s servers or infrastructure.
- Stripe API: The Stripe API allows developers to accept and process payments in their own applications. It provides a set of functions for creating payment forms, charging credit cards, and managing subscriptions, among other things. This allows developers to build e-commerce functionality into their applications without having to build their own payment infrastructure.
Designing an API
There are several tools and languages that you can use to design APIs:
- Programming languages: You can use a wide range of programming languages to design APIs, including:
- C# and VB.NET (for .NET APIs)
- Java (for Android APIs)
- Python (for web-based APIs)
- Ruby (for web-based APIs)
- JavaScript (for web-based APIs)
- Web frameworks: You can use web frameworks to build web-based APIs more easily. Some popular frameworks for building APIs include:
- ASP.NET (for .NET APIs)
- Django (for Python-based APIs)
- Rails (for Ruby-based APIs)
- Express (for JavaScript-based APIs)
- API management platforms: You can use API management platforms to design, deploy, and manage APIs more easily. These platforms often provide features such as documentation, authentication, rate limiting, and monitoring. Some popular API management platforms include:
- Azure API Management
- AWS API Gateway
- Google Cloud Endpoints
- Apigee
- API documentation tools: You can use API documentation tools to automatically generate documentation for your API based on your code. Some popular API documentation tools include:
- Swagger
- Sandcastle (for .NET APIs)
- Doxygen (for C++ APIs)
- Testing tools: You can use testing tools to ensure that your API is working correctly. Some popular testing tools for APIs include:
- Postman (for testing web-based APIs)
- cURL (for testing web-based APIs)
- NUnit (for testing .NET APIs)
- JUnit (for testing Java APIs)
- IDEs: You can use integrated development environments (IDEs) to design and implement your API more easily. Some popular IDEs for API development include:
- Visual Studio (for .NET APIs)
- Eclipse (for Java APIs)
- PyCharm (for Python APIs)
- RubyMine (for Ruby APIs)
- WebStorm (for JavaScript APIs)
Live Session at VIT
At VIT, a Live Session was conducted on designing API using Visual Studio 2022 IDE. Dot.NET Version 7.0 was used with C#.
To design an API using .NET, you can follow these steps:
- Determine the purpose and scope of your API. What kind of data or functionality do you want to expose to external developers? What kinds of applications do you want to enable them to build?
- Decide on the interface for your API. Will it be a web-based API that uses HTTP and HTTPS, or will it be a library API that developers can use in their own .NET applications?
- Define the data structures and object models for your API. What kinds of data will your API need to work with, and how will it be structured? Consider using .NET’s object-oriented features, such as classes and interfaces, to define your data models.
- Implement the API functionality. Write the code for your API, using .NET languages such as C# or VB.NET. Use .NET’s built-in libraries and frameworks, such as ASP.NET and ADO.NET, to implement the various features and functionality of your API.
- Test and debug your API. Use .NET’s debugging tools and test frameworks, such as Visual Studio’s debugger and NUnit, to ensure that your API is working correctly.
- Document your API. Create documentation for your API that explains how it works, what it does, and how developers can use it in their own applications. You can use tools such as Swagger or Sandcastle to automatically generate API documentation from your code.
- Deploy and host your API. Decide on a hosting solution for your API, such as a cloud platform like Azure or AWS, or a dedicated server. Deploy your API to the hosting environment and make it available to external developers.
- Manage and maintain your API. Monitor your API’s usage, handle any issues that arise, and make updates and improvements as needed. Consider implementing features such as authentication and rate limiting to manage access to your API.